How to Use String Templates with Spring AI
0 CommentsLast Updated on October 22, 2024 by jt
A hidden feature of Spring AI is the support of String Templates, aka StringTemplates.
StringTemplate is a lightweight Java template engine. It is simple, and easy to use. StringTemplate is a stable project, established in 2007 and now on its 4 major release.
Here is an example from the homepage of the Stringtemplate website.

On the first line, a new Stringtemplate is defined with the <name>
placeholder. The second line provides a value for the name
placeholder. From the output of the render()
method, you can see how the template is used to create a new String with the given value.
This, of course, is a very simple example the actual capabilities of StringTemplate are much more robust. You can explore the features and capabilities of StringTemplate here.
In the context of Spring AI, StringTemplate is used to aid us in writing prompts. Prompt Engineering is an important and evolving aspect of working with Large Language Models (LLMs). You can learn more about Prompt Engineering in this series of posts, starting here.
Using String Template with Spring AI
This example will build upon the Spring AI project we created in my previous post, Introduction to Spring AI.
The use case for our demo is to ask the LLM to respond with the name of the capital when given a state or country.
First, lets add two Java records to handle the request and response.
GetCapitalRequest.java
public record GetCapitalRequest(String stateOrCountry) {
}
GetCapitalResponse.java
public record GetCapitalResponse(String answer) {
}
In the Spring MVC Controller, QuestionController.java
add the following endpoint. This configures Spring MVC to handle the request and response.
@PostMapping("/capital")
public GetCapitalResponse getCapital(@RequestBody GetCapitalRequest getCapitalRequest) {
return this.openAIService.getCapital(getCapitalRequest);
}
Add the following StringTemplate to your resource/templates
directory.
get-capital-prompt.st
What is the capital of {stateOrCountry}?
Add the following method to the interface OpenAIService.java
.
GetCapitalResponse getCapital(GetCapitalRequest getCapitalRequest);
We can use Spring to autowire the StringTemplate into the implementation of our OpenAI Service. Add the following to OpenAIServiceImpl.java
. Spring will read the file from the resources
directory and inject it into our service at runtime.
@Value("classpath:templates/get-capital-prompt.st")
private Resource getCapitalPrompt;
Now, let’s implement the service method. Add the following method to OpenAIServiceImpl.java
@Override
public GetCapitalResponse getCapital(GetCapitalRequest getCapitalRequest) {
PromptTemplate promptTemplate = new PromptTemplate(getCapitalPrompt);
Prompt prompt = promptTemplate.create(Map.of("stateOrCountry",
getCapitalRequest.stateOrCountry());
ChatResponse response = chatModel.call(prompt);
return new GetCapitalResponse(response.getResult().getOutput().getContent());
}
Here you can see we are using PromptTemplate
with the StringTemplate file. We simply pass a Map to the create
method to bind values to the template. The inner workings of StringTemplate are abstracted from us by Spring AI.
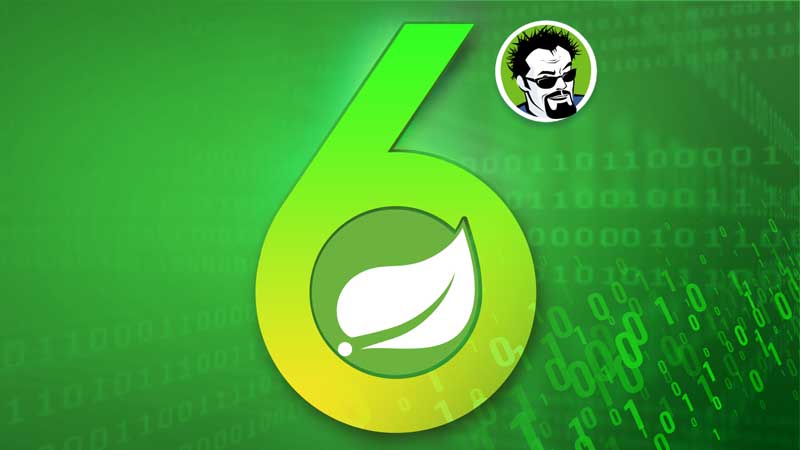
Spring Framework 6: Beginner to Guru
Checkout my best selling course on Spring Framework 6. This is the most comprehensive course you will find on Udemy. All things Spring!
Try It Out in Postman
Post the following JSON to the /capital
endpoint as follows.

This will generate the following response.

We can see that we only provided the State Florida
to the LLM. Using the context provided by the StringTemplate, the LLM received a request asking for the capital.
While this is a very simple example, it demonstrates how easy it is to use StringTemplates when creating prompts in Spring AI. You can easily create much more complex templates with a many values to bind.
You can learn about using Structured Outputs with Spring AI in this next post here.
Spring AI: Beginner to Guru
If you wish to learn much more about Spring AI, check out my Udemy course Spring AI: Beginner to Guru!
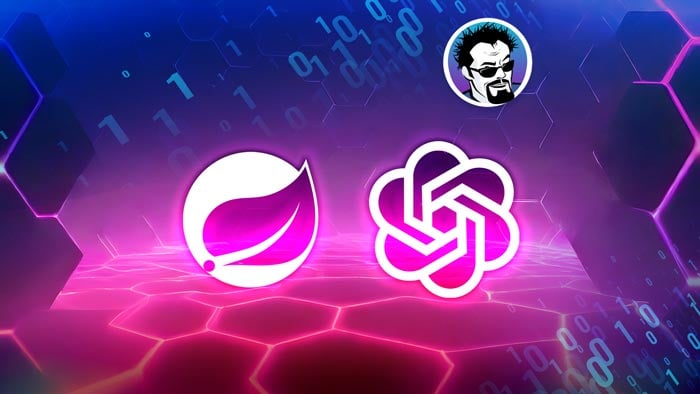