How to Convert String to int in Java
0 CommentsLast Updated on October 22, 2024 by jt
String to int conversions are a common task in Java, especially when dealing with user input or API calls. Java provides several ways to perform this conversion. This blog post will cover the most common methods for converting a String to an Integer and cover best practices to avoid potential errors.
String to int Using Integer.parseInt()
Using Integer.parseInt()
is the most common way to convert a String
into an int
. This method accepts a String value and returns its integer value.
Example:
public class StringToIntExample {
public static void main(String[] args) {
String str = "123";
int i = Integer.parseInt(str);
System.out.println("Converted integer: " + i);
}
}
-----------------output----------------
Converted integer: 123
This is a simple example. This will throw a NumberFormatException
if the input string cannot be parsed as a valid integer.
Following is an example catching the exception:
try {
int i = Integer.parseInt(str);
} catch (NumberFormatException e) {
System.out.println("Invalid input: " + e.getMessage());
}
String to int Number Base Conversion
The Integer.parseInt()
method also supports converting from other number bases (e.g., binary, hexadecimal). You can specify the number base as a second argument.
Here is an String to int example of converting from binary and hexadecimal.
int binary = Integer.parseInt("101", 2); // Binary to decimal
int hex = Integer.parseInt("7F", 16); // Hexadecimal to decimal
Using Integer.valueOf()
Another way to convert a string to an integer is using Integer.valueOf()
. It works similarly to parseInt()
, but instead of returning a primitive int
, it returns an Integer
object (a wrapper class).
Here is an example of using Integer.ValueOf():
Integer number = Integer.valueOf("456");
System.out.println("Integer value: " + number);
Using Integer.decode() for Different Number Formats
We can also use Integer.docode() to convert Strings in different formats to Integers.
For example:
int decimal = Integer.decode("123");
int hex = Integer.decode("0x7F"); // Hexadecimal
int octal = Integer.decode("077"); // Octal
Handling Conversion Safely with Apache Commons
For more robust error handling, you can use libraries like Apache Commons, which provide utility methods that avoid throwing exceptions. These help reduce blocks of verification and exception handling in your code.
Example using Apache Commons:
import org.apache.commons.lang3.math.NumberUtils;
int number = NumberUtils.toInt("789", -1); // Returns -1 if conversion fails
String to Integer Best Practices
- Check for Null Values – Passing a
null
string toparseInt()
orvalueOf()
will throw aNullPointerException
. Always check fornull
before attempting conversion. - Check For Non-Numeric Characters: Strings containing non-numeric characters will cause a
NumberFormatException
. You should validate input to ensure it’s numeric before attempting conversion. - Empty Strings: Attempting to parse an empty string (
""
) will also throw aNumberFormatException
. Ensure the string is not empty before converting. - Consider Using Third Party Libraries – Libraries like Apache Commons or Google Guava can simplify validation and error handling
If you need to convert an int to a String, checkout this post for How to Convert an int to a String in Java!
As always, you can find the source code for this post in my Github repository here.
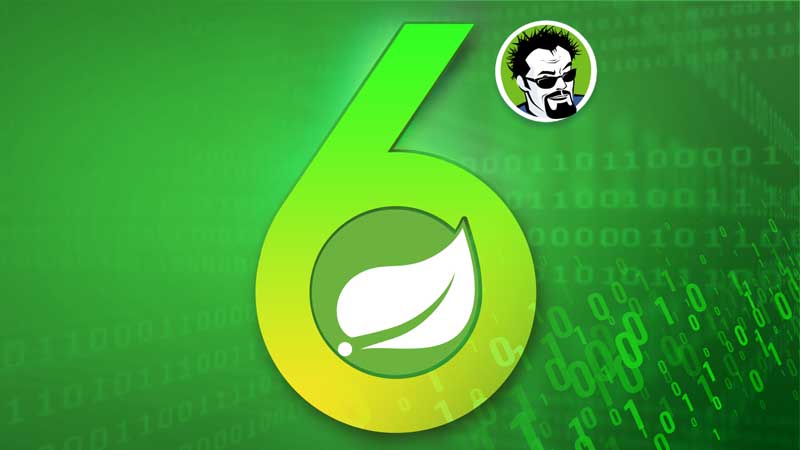
Spring Framework 6: Beginner to Guru
Checkout my best selling course on Spring Framework 6. This is the most comprehensive course you will find on Udemy. All things Spring!