Jackson Dependency Issue in Spring Boot with Maven Build
11 CommentsRecently while working with Jackson within a Spring Boot project, I encountered an issue I’d like to share with you.
Jackson is currently the leading option for parsing JSON in Java. The Jackson library is composed of three components: Jackson Databind, Core, and Annotation. Jackson Databind has internal dependencies on Jackson Core and Annotation. Therefore, adding Jackson Databind to your Maven POM dependency list will include the other dependencies as well. To use the latest Jackson library, you need to add the following dependency in the Maven POM.
. . . <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-databind</artifactId> <version>2.7.4</version> </dependency> . . .
The above dependency works well in other Java projects, but unfortunately in a Spring Boot 1.3.x application, you may stumble upon this error.
You may see several different errors. Here are some additional examples.
java.lang.NoSuchMethodError: com.fasterxml.jackson.annotation.JsonFormat$Value.empty()Lcom/fasterxml/jackson/annotation/JsonFormat$Value; at com.fasterxml.jackson.databind.cfg.MapperConfig.<clinit>(MapperConfig.java:50) at com.fasterxml.jackson.databind.ObjectMapper.<init>(ObjectMapper.java:543) at com.fasterxml.jackson.databind.ObjectMapper.<init>(ObjectMapper.java:460) at guru.springframework.blog.jsonwithjackson.jsonreader.JsonNodeDemo.<init>(JsonNodeDemo.java:19) at guru.springframework.blog.jsonwithjackson.jsonreader.JsonNodeDemoTest.testReadJsonWithJsonNode(JsonNodeDemoTest.java:15) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:497) at org.junit.runners.model.FrameworkMethod$1.runReflectiveCall(FrameworkMethod.java:50) at org.junit.internal.runners.model.ReflectiveCallable.run(ReflectiveCallable.java:12) at org.junit.runners.model.FrameworkMethod.invokeExplosively(FrameworkMethod.java:47) at org.junit.internal.runners.statements.InvokeMethod.evaluate(InvokeMethod.java:17) at org.junit.runners.ParentRunner.runLeaf(ParentRunner.java:325) at org.junit.runners.BlockJUnit4ClassRunner.runChild(BlockJUnit4ClassRunner.java:78) at org.junit.runners.BlockJUnit4ClassRunner.runChild(BlockJUnit4ClassRunner.java:57) at org.junit.runners.ParentRunner$3.run(ParentRunner.java:290) at org.junit.runners.ParentRunner$1.schedule(ParentRunner.java:71) at org.junit.runners.ParentRunner.runChildren(ParentRunner.java:288) at org.junit.runners.ParentRunner.access$000(ParentRunner.java:58) at org.junit.runners.ParentRunner$2.evaluate(ParentRunner.java:268) at org.junit.runners.ParentRunner.run(ParentRunner.java:363) at org.junit.runners.Suite.runChild(Suite.java:128) at org.junit.runners.Suite.runChild(Suite.java:27) at org.junit.runners.ParentRunner$3.run(ParentRunner.java:290) at org.junit.runners.ParentRunner$1.schedule(ParentRunner.java:71) at org.junit.runners.ParentRunner.runChildren(ParentRunner.java:288) at org.junit.runners.ParentRunner.access$000(ParentRunner.java:58) at org.junit.runners.ParentRunner$2.evaluate(ParentRunner.java:268) at org.junit.runners.ParentRunner.run(ParentRunner.java:363) at org.junit.runner.JUnitCore.run(JUnitCore.java:137) at com.intellij.junit4.JUnit4IdeaTestRunner.startRunnerWithArgs(JUnit4IdeaTestRunner.java:119) at com.intellij.junit4.JUnit4IdeaTestRunner.startRunnerWithArgs(JUnit4IdeaTestRunner.java:42) at com.intellij.rt.execution.junit.JUnitStarter.prepareStreamsAndStart(JUnitStarter.java:234) at com.intellij.rt.execution.junit.JUnitStarter.main(JUnitStarter.java:74) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:497) at com.intellij.rt.execution.application.AppMain.main(AppMain.java:144) java.lang.NoClassDefFoundError: Could not initialize class com.fasterxml.jackson.databind.SerializationConfig at com.fasterxml.jackson.databind.ObjectMapper.<init>(ObjectMapper.java:543) at com.fasterxml.jackson.databind.ObjectMapper.<init>(ObjectMapper.java:460) at guru.springframework.blog.jsonwithjackson.jsonreader.ObjectMapperDemo.readJsonWithObjectMapper(ObjectMapperDemo.java:13) at guru.springframework.blog.jsonwithjackson.jsonreader.ObjectMapperDemoTest.testReadJson(ObjectMapperDemoTest.java:13) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:497) at org.junit.runners.model.FrameworkMethod$1.runReflectiveCall(FrameworkMethod.java:50) at org.junit.internal.runners.model.ReflectiveCallable.run(ReflectiveCallable.java:12) at org.junit.runners.model.FrameworkMethod.invokeExplosively(FrameworkMethod.java:47) at org.junit.internal.runners.statements.InvokeMethod.evaluate(InvokeMethod.java:17) at org.junit.runners.ParentRunner.runLeaf(ParentRunner.java:325) at org.junit.runners.BlockJUnit4ClassRunner.runChild(BlockJUnit4ClassRunner.java:78) at org.junit.runners.BlockJUnit4ClassRunner.runChild(BlockJUnit4ClassRunner.java:57) at org.junit.runners.ParentRunner$3.run(ParentRunner.java:290) at org.junit.runners.ParentRunner$1.schedule(ParentRunner.java:71) at org.junit.runners.ParentRunner.runChildren(ParentRunner.java:288) at org.junit.runners.ParentRunner.access$000(ParentRunner.java:58) at org.junit.runners.ParentRunner$2.evaluate(ParentRunner.java:268) at org.junit.runners.ParentRunner.run(ParentRunner.java:363) at org.junit.runners.Suite.runChild(Suite.java:128) at org.junit.runners.Suite.runChild(Suite.java:27) at org.junit.runners.ParentRunner$3.run(ParentRunner.java:290) at org.junit.runners.ParentRunner$1.schedule(ParentRunner.java:71) at org.junit.runners.ParentRunner.runChildren(ParentRunner.java:288) at org.junit.runners.ParentRunner.access$000(ParentRunner.java:58) at org.junit.runners.ParentRunner$2.evaluate(ParentRunner.java:268) at org.junit.runners.ParentRunner.run(ParentRunner.java:363) at org.junit.runner.JUnitCore.run(JUnitCore.java:137) at com.intellij.junit4.JUnit4IdeaTestRunner.startRunnerWithArgs(JUnit4IdeaTestRunner.java:119) at com.intellij.junit4.JUnit4IdeaTestRunner.startRunnerWithArgs(JUnit4IdeaTestRunner.java:42) at com.intellij.rt.execution.junit.JUnitStarter.prepareStreamsAndStart(JUnitStarter.java:234) at com.intellij.rt.execution.junit.JUnitStarter.main(JUnitStarter.java:74) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:497) at com.intellij.rt.execution.application.AppMain.main(AppMain.java:144) java.lang.NoClassDefFoundError: Could not initialize class com.fasterxml.jackson.databind.SerializationConfig at com.fasterxml.jackson.databind.ObjectMapper.<init>(ObjectMapper.java:543) at com.fasterxml.jackson.databind.ObjectMapper.<init>(ObjectMapper.java:460) at guru.springframework.blog.jsonwithjackson.jsonreader.ObjectMapperToMapDemo.readJsonWithObjectMapper(ObjectMapperToMapDemo.java:15) at guru.springframework.blog.jsonwithjackson.jsonreader.ObjectMapperToMapDemoTest.testReadJsonWithObjectMapper(ObjectMapperToMapDemoTest.java:12) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:497) at org.junit.runners.model.FrameworkMethod$1.runReflectiveCall(FrameworkMethod.java:50) at org.junit.internal.runners.model.ReflectiveCallable.run(ReflectiveCallable.java:12) at org.junit.runners.model.FrameworkMethod.invokeExplosively(FrameworkMethod.java:47) at org.junit.internal.runners.statements.InvokeMethod.evaluate(InvokeMethod.java:17) at org.junit.runners.ParentRunner.runLeaf(ParentRunner.java:325) at org.junit.runners.BlockJUnit4ClassRunner.runChild(BlockJUnit4ClassRunner.java:78) at org.junit.runners.BlockJUnit4ClassRunner.runChild(BlockJUnit4ClassRunner.java:57) at org.junit.runners.ParentRunner$3.run(ParentRunner.java:290) at org.junit.runners.ParentRunner$1.schedule(ParentRunner.java:71) at org.junit.runners.ParentRunner.runChildren(ParentRunner.java:288) at org.junit.runners.ParentRunner.access$000(ParentRunner.java:58) at org.junit.runners.ParentRunner$2.evaluate(ParentRunner.java:268) at org.junit.runners.ParentRunner.run(ParentRunner.java:363) at org.junit.runners.Suite.runChild(Suite.java:128) at org.junit.runners.Suite.runChild(Suite.java:27) at org.junit.runners.ParentRunner$3.run(ParentRunner.java:290) at org.junit.runners.ParentRunner$1.schedule(ParentRunner.java:71) at org.junit.runners.ParentRunner.runChildren(ParentRunner.java:288) at org.junit.runners.ParentRunner.access$000(ParentRunner.java:58) at org.junit.runners.ParentRunner$2.evaluate(ParentRunner.java:268) at org.junit.runners.ParentRunner.run(ParentRunner.java:363) at org.junit.runner.JUnitCore.run(JUnitCore.java:137) at com.intellij.junit4.JUnit4IdeaTestRunner.startRunnerWithArgs(JUnit4IdeaTestRunner.java:119) at com.intellij.junit4.JUnit4IdeaTestRunner.startRunnerWithArgs(JUnit4IdeaTestRunner.java:42) at com.intellij.rt.execution.junit.JUnitStarter.prepareStreamsAndStart(JUnitStarter.java:234) at com.intellij.rt.execution.junit.JUnitStarter.main(JUnitStarter.java:74) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:497) at com.intellij.rt.execution.application.AppMain.main(AppMain.java:144)
This error occurs due to Jackson dependency conflict. We are working on a Spring Boot project and it’s inheriting from the Spring Boot parent POM that includes Jackson. Without any Jackson dependency in the project POM, let’s print the Maven dependency tree to view the in-built Jackson dependencies.
mvn dependency:tree -Dincludes=com.fasterxml.jackson.core
The output is this.
[INFO] ------------------------------------------------------------------------ [INFO] Building Blog Posts 0.0.1-SNAPSHOT [INFO] ------------------------------------------------------------------------ [INFO] [INFO] --- maven-dependency-plugin:2.10:tree (default-cli) @ blogposts --- [INFO] guru.springframework:blogposts:jar:0.0.1-SNAPSHOT [INFO] \- com.fasterxml.jackson.core:jackson-databind:jar:2.6.5:compile [INFO] +- com.fasterxml.jackson.core:jackson-annotations:jar:2.6.5:compile [INFO] \- com.fasterxml.jackson.core:jackson-core:jar:2.6.5:compile [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 2.053 s [INFO] Finished at: 2016-05-18T07:32:59-04:00 [INFO] Final Memory: 19M/309M [INFO] ------------------------------------------------------------------------
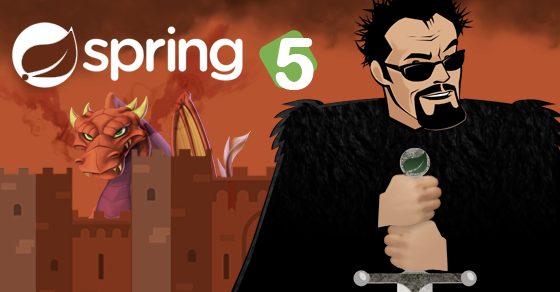
As you can see above, the Spring Boot parent POM uses an older version of Jackson (2.6.5).
Now, if we add the Jackson dependency to our Maven POM using the version like this:
. . . <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-databind</artifactId> <version>2.7.4</version> </dependency> . . .
Maven will pulling in older versions of Jackson-annotation and Jackson-core and overriding the newer ones. We can see this by running the dependency:tree
command again.
[INFO] ------------------------------------------------------------------------ [INFO] Building Blog Posts 0.0.1-SNAPSHOT [INFO] ------------------------------------------------------------------------ [INFO] [INFO] --- maven-dependency-plugin:2.10:tree (default-cli) @ blogposts --- [INFO] guru.springframework:blogposts:jar:0.0.1-SNAPSHOT [INFO] \- com.fasterxml.jackson.core:jackson-databind:jar:2.7.4:compile [INFO] +- com.fasterxml.jackson.core:jackson-annotations:jar:2.6.5:compile [INFO] \- com.fasterxml.jackson.core:jackson-core:jar:2.6.5:compile [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 1.977 s [INFO] Finished at: 2016-05-18T07:35:09-04:00 [INFO] Final Memory: 19M/309M [INFO] ------------------------------------------------------------------------
I did not expect Maven to behave this way in dependency resolution. The POM for the primary Jackson artifact does call for the proper version. However, this seems to be getting overridden by the versions specified explicitly in the Spring Boot parent POM.
Ideally, when working with Spring Boot, is to leverage the curated dependencies in the Spring Boot parent POM. In this case, we drop the version for the Jackson dependency so it will get inherited from the Spring Boot Parent POM.
. . . <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-databind</artifactId> </dependency> . . .
Now, the version will be inherited from the parent POM, and the issue will be resolved.
But what if we want to use a newer version of Jackson? The proper way is to exclude the inherent dependencies, and explicitly add their new versions, like this.
. . . <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-databind</artifactId> <version>2.7.4</version> <exclusions> <exclusion> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-core</artifactId> </exclusion> <exclusion> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-annotations</artifactId> </exclusion> </exclusions> </dependency> <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-core</artifactId> <version>2.7.4</version> </dependency> <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-annotations</artifactId> <version>2.7.4</version> </dependency> . . .
This POM configuration will override the Jackson dependencies set in the Spring Boot parent POM.
This post is specific to Spring Boot version 1.3.3. Naturally, the Spring Boot team will be evolving the version of Jackson used in future releases.
For developers accustomed to working with Maven dependencies, it’s a very easy mistake to include the version in the dependency declaration. This can cause some unintended issues due to version conflicts. It is a little bit of a paradigm shift for experienced developers to depend on the Spring Boot parent POM. Some won’t want to relinquish control, but in the long run I expect you will be better of leveraging the Spring Boot curated dependencies.
If you’d like to learn more about Jackson and Spring Boot checkout my other posts:
sbiyyala
Thank you!
nisckis
Hi,
you can specify a particular version with
2.7.6
the current available maven configuracion properties are available at https://github.com/spring-projects/spring-boot/blob/master/spring-boot-dependencies/pom.xml
Brian Bauman
This was really helpful. All the Stackoverflow articles said to make sure the versions for core and annotations were the same and that wasn’t getting me anywhere. I only wish I found your article sooner
Joaquin Hernandez
Thank you, Was the solution to my problem.
Christian Harms
This also happens with Spring 1.5.9 … thank you for this article.
Scott McCrory
And also with Spring Boot 1.5.11… ditto, thank you for the article!
DaBoo
Thanx for detailed explanation!!!
Nicolas
Nice job! It’s worked fine for me. Thank you for sharing.
Swapnali
Thanks a lot! Perfect explanation and solution, It resolved my issue.
Vinicius Castelano
Thanks! It resolved my issue.
Sarayu
Spent a lot of days on figuring out the cause, You are the saviour!