Configuring Spring Boot for Oracle
21 CommentsWhen you start with Spring Boot, it will automatically support H2 if no other data sources have been defined and H2 is found on the classpath. I’ve been using H2 for development for sometime now. It works very well. Â All modern relational databases are going to support ANSI SQL. But each is going to have its own nuances and extensions. One thing of the things I like about H2 is its Oracle compatibility mode. It allows H2 to act like an Oracle database. It’s not perfect, but it does do a pretty good job.
The Spring Framework is the most popular Java framework used for building enterprise class applications. Oracle is the most popular database used in the enterprise. So chances are, if you are developing Spring Applications, sooner or later, you’re going to be persisting to an Oracle database.
Oracle Database Driver
The Oracle JDBC drivers are not in public Maven repositories due to legal restrictions. This is really rather annoying. Oracle, if you’re reading this – really? Come on, fix this. Please.
So, if you are in a company, chances are you will have a Nexus installation with the Oracle JDBC jar installed. But if you are not, you will need to download the JDBC driver from Oracle (after accepting the terms and conditions you probably won’t read). And then you can install it into your local Maven repository manually.
You can install a JAR into your Maven repository using this Maven command. You may need to adjust the version and name depending on the JDBC driver version you download.
mvn install:install-file -Dfile=ojdbc7.jar -DgroupId=com.oracle -DartifactId=ojdbc7 -Dversion=12.1.0.1 -Dpackaging=jar
Spring Boot Configuration for Oracle
Maven Dependency
You will need to add the Oracle Driver to your Maven (or Gradle) dependencies.
<dependency> <groupId>com.oracle</groupId> <artifactId>ojdbc7</artifactId> <version>12.1.0.1</version> </dependency>
Oracle Datasource
The easiest approach is to create a configuration bean in the package structure of your Spring Boot application. This will create a new Oracle datasource for your Spring Boot application. Once you specify a data source, Spring Boot will no longer create the H2 data source for you automatically.
@Bean DataSource dataSource() throws SQLException { OracleDataSource dataSource = new OracleDataSource(); dataSource.setUser(username); dataSource.setPassword(password); dataSource.setURL(url); dataSource.setImplicitCachingEnabled(true); dataSource.setFastConnectionFailoverEnabled(true); return dataSource; }
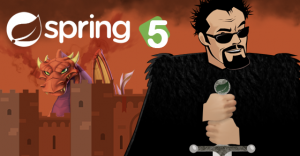
Spring Boot Basic Configuration for Oracle
Spring Boot Properties
Configuring a different datasource in Spring Boot is very simple. When you supply datasource properties in Spring Boot’s application.properties file, Spring Boot will use them to configure the datasource. To configure Spring Boot for Oracle, add the following lines to your properties file.
#Basic Spring Boot Config for Oracle spring.datasource.url= jdbc:oracle:thin:@//spring.guru.csi0i9rgj9ws.us-east-1.rds.amazonaws.com:1521/ORCL spring.datasource.username=system spring.datasource.password=manager spring.datasource.driver-class-name=oracle.jdbc.OracleDriver #hibernate config spring.jpa.database-platform=org.hibernate.dialect.Oracle10gDialect
Spring Boot Advanced Configuration for Oracle
Oracle is a highly advanced and highly configurable RDBMS. There is a reason Oracle is the #1 database in the enterprise. The basic example above will work for just about any JDBC data source you need to configure for use with Spring Boot. They will all have a url, user name, password, and driver class. But with Oracle, there are a number of advanced properties you may need to set. Especially if you’re using Oracle RAC.
Spring Boot will set vendor specific properties using spring.datasource.<property name>. And you absolutely can go this route. However, based on my experience, it might be time to switch to a Java based configuration. Spring Boot will create the data source from just the properties file, or will forgo the automatic data source creation if you’re doing a more traditional method in Spring to define the data source bean.
In this section, I’m going to show you how to use a Spring configuration bean to create the Oracle JDBC datasource.
Oracle Properties
In this example, I’m going to show you how to externalise the Oracle connection properties to a properties file.
In our Spring Boot application.properties file we want to set the following properties.
#Oracle connection oracle.username=system oracle.password=manager oracle.url=jdbc:oracle:thin:@//spring.guru.csi0i9rgj9ws.us-east-1.rds.amazonaws.com:1521/ORCL
Next, on our Configuration class for Oracle, we want to add the following annotation:
@ConfigurationProperties("oracle")
This tells Spring to look for the property prefix of Oracle when binding properties. Now if our configuration class has a property called ‘whatever’, Spring would try to bind the property value of ‘oracle.whatever’ to the property in the configuration class.
Now if we add the following properties to our configuration class, Spring will use them in the creation of our Oracle data source.
@NotNull private String username; @NotNull private String password; @NotNull private String url; public void setUsername(String username) { this.username = username; } public void setPassword(String password) { this.password = password; } public void setUrl(String url) { this.url = url; }
The final Oracle configuration class looks like this:
OracleConfiguration.class
package guru.springframework.configuration; import oracle.jdbc.pool.OracleDataSource; import org.springframework.boot.context.properties.ConfigurationProperties; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.context.annotation.Profile; import javax.sql.DataSource; import javax.validation.constraints.NotNull; import java.sql.SQLException; @Configuration @ConfigurationProperties("oracle") public class OracleConfiguration { @NotNull private String username; @NotNull private String password; @NotNull private String url; public void setUsername(String username) { this.username = username; } public void setPassword(String password) { this.password = password; } public void setUrl(String url) { this.url = url; } @Bean DataSource dataSource() throws SQLException { OracleDataSource dataSource = new OracleDataSource(); dataSource.setUser(username); dataSource.setPassword(password); dataSource.setURL(url); dataSource.setImplicitCachingEnabled(true); dataSource.setFastConnectionFailoverEnabled(true); return dataSource; } }
Hibernate Configuration
We will want to tell Hibernate to use the Oracle dialect. We do this by adding the following property to the Spring Boot application.properties file.
Required
spring.jpa.database-platform=org.hibernate.dialect.Oracle10gDialect
Optional
If you’re used to using the H2 database, database tables will automatically be generated by Hibernate. If you want the same behavior in Oracle, you’ll need to set the ddl-auto property of Hibernate to ‘create-drop’. The Spring Boot documentation has additional information about database initialization. To have tables automatically created in Oracle, set the following property in your application.properties file.
spring.jpa.hibernate.ddl-auto=create-drop
Amazon RDS
In testing the code for this post, I spooled up an Oracle instance using Amazon RDS. This makes creating an Oracle database crazy easy. If you want to test this out yourself, I’ve checked in the code on GitHub here. You can check it out, and setup your own Oracle instance on Amazon RDS. Just update the connection properties in application.properities. I branched the code from my tutorial series on building a web application with Spring Boot. Everything will work – EXCEPT create and save. Oracle handles ID generation a little differently and I did not update the JPA mapping for this.
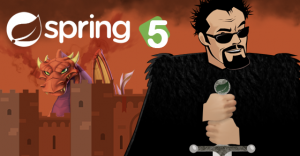
snicoll
Just a quick remark: you don’t need to create all that code to persist to Oracle. Spring Boot supports configuration options for any datasource actually so a) You don’t need to write those properties since we expose these (and much more) in a standard fashion and b) you don’t need to write the datasource bean at all as we detect the presence of these keys (and a jdbc driver) and react accordingly.
See the documentation for more details: http://docs.spring.io/spring-boot/docs/current/reference/htmlsingle/#boot-features-connect-to-production-database
jt
Thanks for pointing this out! I’ll get this post updated.
Vimalraj
For oracle 11g , we need to set dataSource.setConnectionCachingEnabled(true);, then only Fast Connection Failover can ve enabled.
mike
I might be looking at some old docs for Oracle JDBC, but does the use of FCF not require that the ons.jar be in the CLASSPATH (which can be downloaded from Oracle on same page as the JDBC driver) and ONS (Oracle Notification Service) running somewhere on the Oracle server infrastructure (not being a DBA I just don’t know where that would live in a multi-node RAC environment). If true, there might be other changes applicable, such as would the URL format be changed to include each node another pooling parameters, such as:
jdbc:oracle:thin:@(DESCRIPTION=(ADDRESS=(PROTOCOL=TCP)(HOST=host1)
(PORT=1522))(ADDRESS=(PROTOCOL=TCP)(HOST=host2)(PORT=1522))
(LOAD_BALANCE=yes)(CONNECT_DATA=(SERVICE_NAME=RCLB)))
and/or an additional connection property of ONSConfiguration listing node IP:ports.
Sorry for feedback that includes so many questions; However, I’m working through this myself and I’m hoping that together we might find the proper solution quicker (I’ll be sure to share anything new I learn later). But this is some of the first info I’ve found on how to connect to RAC from Spring Boot — I think it is fantastic and it is much appreciated.
Thanks,
Mike
Sangz
The above article is copied from https://dzone.com/articles/configuring-spring-boot-for-oracle or vice versa?
jt
I have an agreement with Dzone to allow them to publish content from my blog.
Eric
please provide the project file structure for this example. Thanks!
Saurav Rath
The last function returns a n OracleDataSource object but the return type is DataSource. Please fix this
Bernie
@Saurav OracleDataSource implements DataSource, and it’s better to return the interface than the concrete class. What needs to be fixed?
Suprem3_Lady
Hello Jt, Your tutorials are awesome.
When i follow above procedure, i get the below error. could you please help me out in this
“org.springframework.beans.factory.BeanCreationException: Error creating bean with name ‘entityManagerFactory’ defined in class path resource [org/springframework/boot/autoconfigure/orm/jpa/HibernateJpaAutoConfiguration.class]: Invocation of init method failed; nested exception is org.hibernate.service.spi.ServiceException: Unable to create requested service [org.hibernate.engine.jdbc.env.spi.JdbcEnvironment]”
Phil
Why in application.properties both
spring.datasource.url=
spring.datasource.username=
spring.datasource.password=
AND
oracle.username=
oracle.password=
oracle.url=
are defined?
Why does one need both? Thanks
Nicolas Alberto Guevara
Buenos dias, segui el tutorial y cuando ejecuto mi proyecto me da error:
org.springframework.beans.factory.BeanCreationException: Error creating bean with name ‘entityManagerFactory’ defined in class path resource [org/springframework/boot/autoconfigure/orm/jpa/HibernateJpaAutoConfiguration.class]: Invocation of init method failed; nested exception is javax.persistence.PersistenceException: [PersistenceUnit: default] Unable to build Hibernate SessionFactory
Hash
I am getting the following error :
java.sql.SQLException: Invalid Oracle URL specified: OracleDataSource.makeURL
Code i used is :
Connection con = new OracleConfiguration ().dataSource().getConnection();
# Properties file
oracle.url=jdbc:oracle:thin:@//localhost:1521/orcl
oracle.username=admin
oracle.password=admin
Can you help me out with this, how did i open a connection
Hicham
try oracle.url=jdbc:oracle:thin:@localhost:1521:orcl
Alex
Hi there, thanks for the great write up!
I was handed over a project to maintain that has an exact implementation for the Oracle data source as the one mentioned in the article.
I was now informed that the app does thousands of logons/min to the database when a certain importer is running and was suggested by our DBA’s that we change the logic to run through an already connected connection pool to reduce the stress to the database server.
Can this be done in OracleConfiguration class when setting up the dataSource bean?
Thanks in advance and sorry for the basic question but i am obviously missing something here that keeps me from figuring this out 🙂
Faridoon Noori
Hi,
I am using Oracle 11g ,and I got error when I added that in pom.xml !?1
Do you know what can be the reason?
Faridoon
niez
what if we need a SECURITY SSL_SERVER_CERT_DN
Michael Holopainen
This instruction is completely missing both UCP and how to specify the Schema for the connection .
Gerald Venzl
Hey John,
Great article about configuring Spring Boot with Oracle Database.
As to your comment:
The Oracle JDBC drivers are not in public Maven repositories due to legal restrictions. This is really rather annoying. Oracle, if you’re reading this – really? Come on, fix this. Please.
This actually has been fixed, you can find the official JDBC drivers and more under the “com.oracle.database.jdbc” group: https://search.maven.org/search?q=com.oracle.database.jdbc
It would be great if you could update this article to reflect this.
Thanks,
Gerald
Gerald Venzl
Hi John,
Thanks for this great article about Spring Boot with Oracle Database.
As to your point: “The Oracle JDBC drivers are not in public Maven repositories due to legal restrictions. This is really rather annoying. Oracle, if you’re reading this – really? Come on, fix this. Please.”
The official Oracle JDBC drivers are available on Maven Central. They can be found under: https://search.maven.org/search?q=com.oracle.database.jdbc
It would be great if you could update your article to reflect these changes, please.
Thanks,
Gerald