Mastering Jackson Annotations: A Guide to Simplified JSON Mapping for Java
4 CommentsLast Updated on October 22, 2024 by jt
Jackson is a popular library in Java used for processing JSON data. It provides annotations that allow developers to customize how Java objects are serialized into JSON and how JSON data is deserialized into Java objects.
Jackson Annotations are an import aspect to controlling how Jackson creates and consumes JSON payloads. In this post, we will explore how you can use Jackson annotations for the processing of JSON messages.
Types of Jackson Annotations
Jackson Annotations can be broken into four different categories.
- General Annotations – These annotations apply to both reading and writing JSON.
- Serialization Annotations – These annotations control how Java objects are converted into JSON.
- Deserialization Annotations – Annotations which control how JSON is converted into Java objects.
- Polymorphic Type Annotations – Annotations which assist with polymorphic types.
Jackson General Annotations
- @JsonProperty
- @JsonIgnore
- @JsonIgnoreProperties
- @JsonIgnoreType
- @JsonAutoDetect
@JsonUnwrapped
@JsonView
@JsonManagedReference
and@JsonBackReference
@JsonIdentityInfo
@JsonFilter
@JsonPropertyDescription
@JsonProperty
The @JsonProperty
annotation is used to map property names with JSON keys during serialization and deserialization. By default, if you try to serialize a POJO, the generated JSON will have keys mapped to the fields of the POJO. If you want to override this behavior, you can use the @JsonProperty
annotation on the fields. It takes a String
attribute that specifies the name that should be mapped to the field during serialization.
You can also use @JsonProperty annotation during deserialization when the property names of the JSON and the field names of the Java object do not match.
@JsonIgnore
The @JsonIgnore
annotation marks a field in a POJO to be ignored by Jackson during serialization and deserialization. Jackson ignores the field in both JSON serialization and deserialization. An example of Java class that uses the @JsonIgnore
annotation is this.
@JsonIgnoreProperties
The @JsonIgnoreProperties
annotation is used at the class level to ignore fields during serialization and deserialization. The properties that are declared in this annotation will not be mapped to the JSON content.
@JsonIgnoreType
The @JsonIgnoreType
annotation is used to mark a class to be ignored during serialization and deserialization. It marks all the properties of the class to be ignored while generating and reading JSON. An example of Java class that uses the @JsonIgnoreType
annotation is this.
Example Using @JsonProperty, @JsonIgnore, @JsonIgnoreProperties and @JsonIgnoreType
Jackson offers a lot of control over what properties are or are not included in serialization. Consider the two following classes, note the Jackson Annotations being used.
@Data
@Builder
@AllArgsConstructor
@JsonIgnoreType
public static class Address {
private String addressLine1;
private String city;
private String state;
private String zip;
}
@Data
@Builder
@AllArgsConstructor
@JsonIgnoreProperties({"ssn"})
public static class Person {
private String firstName;
@JsonProperty("surname")
private String lastName;
private String phoneNumber;
@JsonIgnore
private LocalDate birthDate;
private String ssn;
private String email;
private BigDecimal salary;
private Address address;
}
We can test the serialization functionality with the following test:
@Test
void testJsonProperties() throws JsonProcessingException {
val person = Person.builder()
.firstName("John")
.lastName("Doe")
.phoneNumber("123-456-7890")
.birthDate(LocalDate.of(1970, 1, 1))
.ssn("123-45-6789")
.email("exmaple.com")
.salary(new BigDecimal("75000.00"))
.address(Address.builder()
.addressLine1("1234 Some Street")
.city("Some City")
.state("ST")
.zip("12345")
.build())
.build();
ObjectMapper objectMapper = new ObjectMapper();
System.out.println(objectMapper.writeValueAsString(person));
}
----------------------------------------------------------
output:
{"firstName":"John","phoneNumber": "123-456-7890","email":"exmaple.com","salary":75000.00,"surname":"Doe"}
Note the following:
- The
lastName
property has been renamed tosurname
using the@JsonProperty
annotation birthDate
is not displayed due to the@JsonIgnore
annotationssn
is not displayed due to the@JsonIgnoreProperties
annotationaddress
is omitted due to the @JsonIgnoreType annotation
If you are not familar with using the Jackson objectMapper see this blog post.
@JsonAutoDetect
The @JsonAutoDetect
annotation is used at the class level to tell Jackson to override the visibility of the properties of a class during serialization and deserialization. You can set the visibility with the following elements:
creatorVisibility
fieldVisibility
getterVisibility
setterVisibility
isGetterVisibility
The JsonAutoDetect
class defines public static constants that are similar to Java class visibility levels. They are:
ANY
DEFAULT
NON_PRIVATE
NONE
PROTECTED_AND_PRIVATE
PUBLIC_ONLY
@JsonUnwrapped
The @JsonUnwrapped
annotation unwraps the values during serialization and deserialization. It helps in rendering the values of a composed class as if they belonged to the parent class. Let us consider an example of Java class that uses the @JsonUnwrapped
annotation.
@JsonView
The @JsonView
annotation is used to include or exclude a property dynamically during serialization and deserialization, and tells the view in which the properties are rendered. Let us consider an example Java class that uses the @JsonView
annotation with Public
and Internal
views.
@JsonManagedReference and @JsonBackReference
The @JsonManagedReference
and @JsonBackReference
annotation are used to create JSON structures that have a bidirectional relationship. Without this annotation, you get an error like this.
"com.fasterxml.jackson.databind.JsonMappingException:Infinite recursion (StackOverflowError)"
Let us consider an example Java class that uses the @JsonManagedReference
and @JsonBackReference
annotations.
d with @JsonManagedReference
is the forward reference which will be included during serialization. The field marked with @JsonBackReference
is the back reference and is usually omitted during serialization.
@JsonIdentityInfo
The @JsonIdentityInfo
tells Jackson to perform serialization or deserialization using the identity of the object. This annotation works similar to the @JsonManagedReference
and @JsonBackReference
annotations with the difference that @JsonIdentityInfo
includes the back reference object.
As you can see, the output gives the information about the employee with his manager details and information about the employees under the manager.
@JsonPropertyDescription
The @JsonPropertyDescription
annotation allows you to add metadata about the field, which will be included in the metadata when a JSON schema is generated from the class. This is a handy way to add the description when you are working with Structured Outputs and Spring AI.
Jackson Serialization Annotations
Jackson provides several annotations that you can use in POJO’s to serialize Java objects to JSON. These annotations are:
@JsonValue
@JsonInclude
@JsonGetter
@JsonAnyGetter
@JsonPropertyOrder
- @JsonFormat
@JsonRawValue
@JsonSerialize
@JsonRootName
@JsonAppend
- @JsonNaming
@JsonValue
The @JsonValue
annotation is used at the method level. This annotation tells Jackson to use this method to generate the JSON string from the Java object.
Typically, if you want to print a serialized object, you override the toString()
method. But, by using the @JsonValue
annotation, you can define the way in which the Java object is to be serialized.
Note: Jackson omits any quotation marks inside the String that is returned by the custom serializer.
@JsonInclude
The @JsonInclude
annotation is used to exclude properties or fields of a class under certain conditions. This is defined using the JsonInclude.Include
enum. This enum contains constants, that determine whether or not to exclude the property. The constants are:
ALWAYS
NON_DEFAULT
NON_EMPTY
NON_NULL
@JsonGetter
The @JsonGetter
annotation is used to customize the generated JSON keys. This is accomplished with the value argument of @JsonGetter
. The value passed is the name that should be used as the JSON key.
@JsonAnyGetter
The @JsonAnyGetter
annotation can be used when you don’t want to declare a property or a method for every possible key in JSON. This annotation is used on the getter methods, which enables you to use a Map
to hold all your properties that you want to serialize.
@JsonPropertyOrder
The @JsonPropertyOrder
annotation tells Jackson to serialize the Java object to JSON, in the order specified as the arguments of the annotation. This annotation also allows partial ordering. The properties are first serialized in the order, in which they are found. Followed by any other properties not included in the annotation.
@JsonFormat
The @JsonFormat
annotation is used to tell Jackson that the format in which the value for a field is serialized. It specifies the format using the JsonFormat.Shape
enum.
Let us consider an example Java class that uses the @JsonFormat
annotation to modify the Date and Time format of an activeDate
field.
@JsonRawValue
The @JsonRawValue
annotation is used on methods and fields. It tells Jackson to serialize the field or property as declared. For example, if you have a String field in your Java class, the JSON value that Jackson generates is enclosed within quotes (” “). But when you annotate the field with @JsonRawValue
, Jackson omits the quotes.
@JsonSerialize
The @JsonSerialize
annotation is used tell Jackson to use the declared custom serializer during the serialization of the field, which is marked with this annotation. Let us consider a POJO that uses the @JsonSerialize
annotation.
@JsonRootName
The @JsonRootName
annotation can be used to tell Jackson to wrap the object to be serialized with a top-level element. You can pass the name as a parameter to the @JsonRootName
annotation. Let us consider that you want to wrap your serialized Java object with the key user
.
@JsonFilter
The @JsonFilter
annotation is used to tell Jackson to use a custom defined filter to serialize the Java object. To define your filter, you need to use the FilterProvider
class. This provider gets the actual filter instance to use. The filter is then configured by assigning the FilterProvider
to ObjectMapper
.
@JsonAppend
The @JsonAppend annotation is used to add virtual properties to the generated JSON during serialization. Typically this is some type of metadata, such as version, which you wish to provide to the consumer.
@JsonNaming
The @JsonNaming Annotation is a class level annotation you can use to set the naming strategy and override the value configured in the ObjectMapper
. This is handy to use when you are using Spring Boot and need to override the autoconfigured ObjectMapper
for a one-off use case.
Jackson library provides four built-in property naming strategies:
- KEBAB_CASE: Name elements are separated by hyphens, e.g. kebab-case.
- LOWER_CASE: All letters are lowercase with no separators, e.g. lowercase.
- SNAKE_CASE: All letters are lowercase with underscores as separators between name elements, e.g. snake_case.
- UPPER_CAMEL_CASE: All name elements, including the first one, start with a capitalized letter, followed by lowercase ones and there are no separators, e.g. UpperCamelCase.
Deserialization Annotations
Let us explore the JSON annotations that can be used to control deserialization of JSON into POJOs. The Jackson deserialization annotations are:
@JsonSetter
@JsonAnySetter
@JsonCreator
@JacksonInject
@JsonDeserialize
@JsonPOJOBuilder
@JsonSetter
The @JsonSetter
annotation tells Jackson to deserialize the JSON into Java object using the name given in the setter method. Use this annotation when your JSON property names are different to the fields of the Java object class, and you want to map them.
As you can see, the JSON to be serialized has a property id
. But no field in the POJO matches this property. Now how will Jackson read this JSON? Here is where the @JsonSetter
annotation can be used to map the property id
to the field personId
. This annotation instructs Jackson to use a setter method for a given JSON property.
@JsonAnySetter
The @JsonAnySetter
annotation is used on setter methods of a Map
field. Sometimes, you may find some JSON values that cannot be mapped to the fields in the Java object class. In such a case, the @JsonAnySetter
captures the data and stores them in a Map
.
@JsonCreator
The @JsonCreator
annotation tells Jackson that the JSON properties can be mapped to the fields of a constructor of the POJO. This is helpful when the JSON properties do not match with the names of the Java object field names. The @JsonCreator
annotation can be used where @JsonSetter
cannot be used. For example, immutable objects which need their initial values to be injected through constructors.
@JacksonInject
The @JacksonInject
annotation is used to tell Jackson that particular values of the deserialized object will be injected and not read from the JSON string.
@JsonDeserialize
The @JsonDeserialize
annotation tells Jackson to use a custom deserializer while deserializing the JSON to Java object. To do so, you need to annotate the field to which you need to apply the custom deserializer.
A Java class that uses the @JsonDeserialize
annotation is this.
Here, the CustomDateDeserializer
class extends the StdDeserializer
class with a generic type Date
. The overriden deserialize()
method returns the Date
object.
@JsonPOJOBuilder
The @JsonPOJOBuilder
annotation instructs Jackson to use a builder to create the bean during deserialization.
Jackson Polymorphic Type Annotations
Jackson’s Polymorphic Type Annotations include:
@JsonTypeInfo
@JsonSubTypes
@JsonTypeName
@JsonTypeId
@JsonTypeIdResolver
@JsonTypeInfo
The @JsonTypeInfo
annotation what type information to include in serialization.
@JsonSubTypes
The @JsonSubType
annotation defines the sub types for the given type.
@JsonTypeName
The @JsonTypeName
annotation defines the name of defined subtypes.
@JsonTypeId
The @JsonTypeId
annotation is used on a property to identify the property should be used as the id value during serialization. The polymorphic metadata is used during deserialization to recreate objects of the same subtypes as they were before serialization
@JsonTypeIdResolver
The @JsonTypeIdResolver annotation is used to identify custom type identity in serialization and deserialization.
You can download the source code of this post from here.
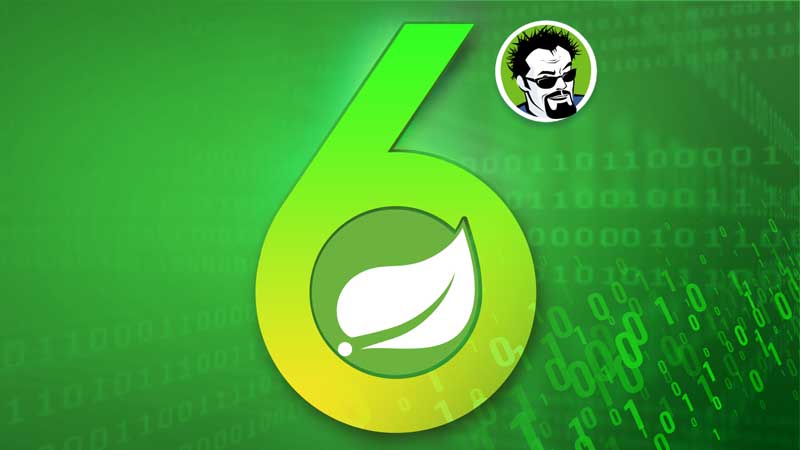
Spring Framework 6: Beginner to Guru
Checkout my best selling course on Spring Framework 6. This is the most comprehensive course you will find on Udemy. All things Spring!
simonmichel
Your code in sample “AutoDetectDemoBean.java” is missing.
Best
shivani
nice writeup, from where we can download source code for the examples in your blog..
Nelson Visbal
In the first example “productId” doesn’t exists in the “IgnoreDemoBean.java” class
@Test
public void testSerializingWithJsonIgnore()
throws JsonProcessingException {
String jsonString = objectMapper.writeValueAsString(new IgnoreDemoBean());
System.out.println(jsonString);
assertThat(jsonString, containsString(“James Clark”));
assertThat(jsonString, not(containsString(“productId”)));
}
Manideep kumar
@JsonAnyGetter anno is un neccesarily creating duplicate elements, whereas if u delete this anno and if u just keep map it is perfectly working fine without any duplicate elements
result:– here in below object city and district are duplicated again
{
“hm” : {
“city” : “kavali”,
“district” : “Nellore”
},
“city” : “kavali”,
“district” : “Nellore”
}
result after deleting the annotation– see here no duplicate elements
{
“hm” : {
“city” : “kavali”,
“district” : “Nellore”
}
}
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.ANY)
public class JsonAnyGetterDemo {
Map hm=Map.of(“city”,”kavali”,”district”,”Nellore”);
@JsonAnyGetter
public Map getHm() {
return hm;
}
public static void main(String[] args) throws Exception{
ObjectMapper objectMapper=new ObjectMapper();
System.out.println(objectMapper.writeValueAsString(new JsonAnyGetterDemo()));
}
}