How to Create Dynamic Output with the Java printf() Method
0 CommentsLast Updated on October 22, 2024 by jt
The Java printf()
output method is used to generate a formatted string. Data from the arguments is written and formatted placeholders. In this tutorial we will explore the functionality of the printf()
method.
The Java language was heavily inspired by the C programming language. As such, the printf()
method is very similar in functionality to C’s printf
method. The syntax and functionality is similar, but not identical to it’s C based inspiration.
printf() Method Signatures
The Java printf() method has three signatures.
System.out.printf(string);
– Applies no formatting, works like theprintln()
method.System.out.printf(format, arguments);
– Accepts a format string, arguments (one or more values)System.out.printf(locale, format, arguments);
– Locale is the first parameter, to allow localization of the output.
The Java printf() method is a convenience method for the Java class java.util.Formatter
.
Frequently, the Formatter class is used with a StringBuilder as follows:
StringBuilder sb = new StringBuilder();
Formatter formatter = new Formatter(sb);
formatter.format("Spring Framework %s", "Guru");
System.out.println(formatter);
Spring Framework Guru
Using printf()
we can see the same functionality.
System.out.printf("Spring Framework %s", "Guru");
Spring Framework Guru
In the above examples, we are using the Format Specifier %s
(String) which gets substituted with the first argument (“Guru”).
The Format Specifiers accept arguments positionally by default.
If we run the following:
System.out.printf("Spring Framework %s ", "Guru");
System.out.printf("%s %s %s", "Spring", "Framework", "Guru");
The output is:
Spring Framework Guru Spring Framework Guru
In this case, we can see the output of the two statements is on the same line. This is because unlike println()
, the printf()
does not append a new line character to the end of the output.
We can resolve this by adding new line escape character (\n
) to the first statement.
System.out.printf("Spring Framework %s \n", "Guru");
System.out.printf("%s %s %s", "Spring", "Framework", "Guru");
We can see that this produces the output on two lines now.
Spring Framework Guru
Spring Framework Guru
We can achieve the same effect using the Format Specifier %n
too.
While the default behavior is to accept the arguments in the order they were passed, we can also reference them by position. Using x$
in the Format Specifier allows us to specify the position of the desired argument.
For example we can reverse the position of the words as follows:
System.out.printf("Spring Framework %s %n", "Guru");
System.out.printf("%s %s %s %n", "Spring", "Framework", "Guru");
System.out.printf("%3$s %2$s %1$s", "Spring", "Framework", "Guru");
This produces:
Spring Framework Guru
Spring Framework Guru
Guru Framework Spring
The Format Specifies follow the following syntax:
%[argument_index$][flags][width][.precision]specifier
The above syntax becomes more relevant as we use more complex types such as Dates.
[argument_index$]
– Position of the argument[flags]
– used to modify the output. Common for formatting integers and floating point numbers[width]
– Field width for outputting the argument. It represents the minimum number of charters written to the output[.precision]
Java Format Specifiers
These are the available Format Specifiers in Java.
- %a or %A Floating point hexadecimal
- %c Unicode character
- %b or %B Boolean
- %d decimal (integer) number (base 10)
- %e or %E exponential floating-point number
- %f floating-point number
- %h or %H Hash code of argument
- %i integer (base 10)
- %n line separator
- %o octal number (base 8)
- %s String
- %S String to Uppercase
- %u unsigned decimal (integer) number
- %x or %X number in hexadecimal (base 16)
- %t or %T formats date/time
- %% print a percent sign
- \% print a percent sign
Java Format Flags
Flag | General | Char | Integral | Floating Pt | Date/ Time | Description |
– | y | y | y | y | y | The result will be left-justified. |
# | y | y | y | The result should use a conversion-dependent alternate form | ||
+ | y | y | The result will always include a sign | |||
‘ ‘ | y | y | The result will include a leading space for positive values | |||
‘0’ | y | y | The result will be zero-padded | |||
‘,’ | y | y | The result will include locale-specific grouping separators | |||
‘(‘ | y | y | The result will enclose negative numbers in parentheses |
Java Escape Characters
The following escape characters are available with printf()
- \b backspace
- \\ backslash
- \f next line first character starts to the right of current line last character
- \n newline
- \r carriage return
- \u Unicode
- \t tab
String Formatting
We’ve previously looked at several String formatting options earlier in this post. We do have several other options we can use with String values.
%S
– String to uppercase- Left Justification Flag
- Output width
We can demonstrate these with the following:
System.out.printf("%s %S %S %n", "Spring", "Framework", "Guru");
System.out.printf("%10s %s %s %n", "Spring", "Framework", "Guru");
System.out.printf("%-10s %s %s %n", "Spring", "Framework", "Guru");
This will produce the following output:
Spring FRAMEWORK GURU
Spring Framework Guru
Spring Framework Guru
You can see in the first line %S
changed the last two words to uppercase.
Using %10s
changed the size of the output to 10 characters, padding on the left.
Using %-10s
changed the size of the output to 10 characters, String is left justified.
Boolean Formatting
The Boolean format specifier will return false if the value evaluates to false or is null. If the argument has a value, and is not a boolean false, it will return true.
The following statements demonstrate the boolean functionality.
System.out.printf("%b%n", null);
System.out.printf("%B%n", null);
System.out.printf("%B%n", true);
System.out.printf("%B%n", Boolean.FALSE);
System.out.printf("%b%n", 2024);
System.out.printf("%b%n", "Java Rocks!");
System.out.printf("%B%n", "JAVA ROCKS!");
System.out.printf("%b%n", LocalDateTime.now());
Produces the following output:
false
FALSE
TRUE
FALSE
true
true
TRUE
true
Character Formatting
Using %c
will output a unicode character.
We can demonstrate the character formatting functionality with the examples below.
System.out.printf("%c%n", 'g');
System.out.printf("%C%n", 'g');
System.out.printf("%C%c%c%c%n", 71, 117, 114, 117);
System.out.printf("%c%c%c%c%n", '\u0047', '\u0075', '\u0072', '\u0075');
This will produce the following output:
g
G
Guru
Guru
You can see in the output we can use a character literal, unicode decimal, or escaped unicode value.
Integer Formatting
We can use the %d
Format Specifier to work with integer values.
In the below example, we can see a demonstration of Integer formatting.
System.out.printf("My Integer: %d%n", 20000L);
System.out.printf(Locale.US, "%,d %n", 20000);
System.out.printf(Locale.GERMAN, "%,d %n", 20000);
System.out.printf(Locale.FRENCH, "%,d %n", 20000);
These statements will produce the following output:
My Integer: 20000
20,000
20.000
20 000
Note how by passing the Locale, the separator changes to the country specific value.
Float and Double Formatting
For float and double values, we can use the %f
Format Specifier. In the examples below, we pass different width and precision values to change the output. We can also use the %e
Format Specifier to format the float value to scientific notation.
We can demonstration the formatting of floats and doubles with the following statements.
System.out.printf("%f%n", Math.PI);
System.out.printf("%20.2f%n", Math.PI);
System.out.printf("%20.18f%n", Math.PI);
System.out.printf("%5.2e%n", Math.PI);
These statements will produce the following output.
3.141593
3.14
3.141592653589793000
3.14e+00
Time Formatting
For time values, we can use the %t
or %T
Format Specifier. These work in conjunction with conversion characters to extract and format portions of the time value.
Conversion | Description |
‘H’ | Hour of the day for the 24-hour clock, formatted as two digits with a leading zero as necessary i.e. 00 - 23 . |
‘I’ | Hour for the 12-hour clock, formatted as two digits with a leading zero as necessary, i.e. 01 - 12 . |
‘k’ | Hour of the day for the 24-hour clock, i.e. 0 - 23 . |
‘l’ | Hour for the 12-hour clock, i.e. 1 - 12 . |
‘M’ | Minute within the hour formatted as two digits with a leading zero as necessary, i.e. 00 - 59 . |
‘S’ | Seconds within the minute, formatted as two digits with a leading zero as necessary, i.e. 00 - 60 (“60 ” is a special value required to support leap seconds). |
‘L’ | Millisecond within the second formatted as three digits with leading zeros as necessary, i.e. 000 - 999 . |
‘N’ | Nanosecond within the second, formatted as nine digits with leading zeros as necessary, i.e. 000000000 - 999999999 . |
‘p’ | Locale-specific morning or afternoon marker in lower case, e.g.”am ” or “pm “. Use of the conversion prefix 'T' forces this output to upper case. |
‘z’ | RFC 822 style numeric time zone offset from GMT, e.g. -0800 . This value will be adjusted as necessary for Daylight Saving Time. For long , Long , and Date the time zone used is the default time zone for this instance of the Java virtual machine. |
‘Z’ | A string representing the abbreviation for the time zone. This value will be adjusted as necessary for Daylight Saving Time. For long , Long , and Date the time zone used is the default time zone for this instance of the Java virtual machine. The Formatter’s locale will supersede the locale of the argument (if any). |
‘s’ | Seconds since the beginning of the epoch starting at 1 January 1970 00:00:00 UTC, i.e. Long.MIN_VALUE/1000 to Long.MAX_VALUE/1000 . |
‘Q’ | Milliseconds since the beginning of the epoch starting at 1 January 1970 00:00:00 UTC, i.e. Long.MIN_VALUE to Long.MAX_VALUE . |
‘R’ | Time formatted for the 24-hour clock as "%tH:%tM" |
‘T’ | Time formatted for the 24-hour clock as "%tH:%tM:%tS" . |
‘r’ | Time formatted for the 12-hour clock as "%tI:%tM:%tS %Tp" . The location of the morning or afternoon marker ('%Tp' ) may be locale-dependent. |
We have a lot of versatility for formatting time values. The code below demonstrates some of the time formatting capabilities.
Date date = new Date();
System.out.println("Use t or T, same result");
System.out.printf("%tT%n", date);
System.out.printf("%TT%n%n", date);
System.out.println("Show time in 12 hour format");
System.out.printf("%tr%n%n", date);
System.out.println("Build Date by position");
System.out.printf("time is %tH:%tM:%tS%n%n", date, date, date);
System.out.println("Show time in 12 hour format, remove additional arguments by referencing index");
System.out.printf("%1$tH:%1$tM:%1$tS %1$tp %1$tL %1$tN %1$tz %n", date);
These statements produce the following output:
Use t or T, same result
14:06:22
14:06:22
Show time in 12 hour format
02:06:22 PM
Build Date by position
time is 14:06:22
Show time in 12 hour format, remove additional arguments by referencing index
14:06:22 pm 900 900000000 -0400
Note in the last example, we only pass one argument, and then reference it by index. We can also use <
to use the previous argument.
For example:
Date date = new Date();
System.out.println("Show time in 12 hour format, remove additional arguments by referencing index");
System.out.printf("%1$tH:%1$tM:%1$tS %1$tp %1$tL %1$tN %1$tz %n", date);
System.out.println("Same Result as above, but using < instead of $");
System.out.printf("%1$tH:%<tM:%<tS %<tp %<tL %<tN %<tz %n", date);
These statements produce the following output:
Show time in 12 hour format, remove additional arguments by referencing index
14:52:56 pm 105 105000000 -0400
Same Result as above, but using < instead of $
14:52:56 pm 105 105000000 -0400
Date Formatting
For date values, we also use the %t
or %T
Format Specifier, but with different Conversion characters.
The following conversion characters are used for formatting Date values.
Conversion | Description |
‘B’ | Locale-specific full month name, e.g. "January" , "February" . |
‘b’ | Locale-specific abbreviated month name, e.g. "Jan" , "Feb" . |
‘h’ | Same as 'b' . |
‘A’ | Locale-specific full name of the day of the week, e.g. "Sunday" , "Monday" |
‘a’ | Locale-specific short name of the day of the week, e.g. "Sun" , "Mon" |
‘C’ | Four-digit year divided by 100 , formatted as two digits with leading zero as necessary, i.e. 00 - 99 |
‘Y’ | Year, formatted as at least four digits with leading zeros as necessary, e.g. 0092 equals 92 CE for the Gregorian calendar. |
‘y’ | Last two digits of the year, formatted with leading zeros as necessary, i.e. 00 - 99 . |
‘j’ | Day of year, formatted as three digits with leading zeros as necessary, e.g. 001 - 366 for the Gregorian calendar. |
‘m’ | Month, formatted as two digits with leading zeros as necessary, i.e. 01 - 13 . |
‘d’ | Day of month, formatted as two digits with leading zeros as necessary, i.e. 01 - 31 |
‘e’ | Day of month, formatted as two digits, i.e. 1 - 31 . |
‘D’ | Date formatted as "%tm/%td/%ty" . |
‘F’ | ISO 8601 complete date formatted as "%tY-%tm-%td" . |
‘c’ | Date and time formatted as "%ta %tb %td %tT %tZ %tY" , e.g. "Sun Jul 20 16:17:00 EDT 1969" . |
The following statements demonstrate date formatting:
Date date = new Date();
System.out.printf("%1$tA, %1$tB %1$tY %n", date);
System.out.printf("%1$td/%1$tm/%1$ty %n", date);
System.out.printf("%1$td/%1$tm/%1$tY %n", date);
System.out.printf("%s %tB %<te, %<tY%n", "Current date: ", date);
System.out.printf("%s %tb %<te, %<ty%n", "Current date: ", date);
System.out.printf("%tD%n", date);
These statements produce the following output:
Wednesday, October 2024
16/10/24
16/10/2024
Current date: October 16, 2024
Current date: Oct 16, 24
10/16/24
Datetime Formatting
For datetime values, we also use the %t
or %T
Format Specifier. We can use a combination of the conversion characters we’ve looked at for time and date.
There are a couple conversion characters available for datetime formatting. The values are as follows:
Conversion | Description |
‘F’ | ISO 8601 complete date formatted as "%tY-%tm-%td" . |
‘c’ | Date and time formatted as "%ta %tb %td %tT %tZ %tY" , e.g. "Sun Jul 20 16:17:00 EDT 1969" . |
The following statements demonstrate the formatting of Datetime values.
Date date = new Date();
System.out.printf("%tF %n", date);
System.out.printf("%tc %n", date);
System.out.printf(Locale.US, "%tc %n", date);
System.out.printf(Locale.UK, "%tc %n", date);
System.out.printf(Locale.GERMAN, "%tc %n", date);
System.out.printf(Locale.CHINESE, "%tc %n", date);
These statements generate the following output:
2024-10-16
Wed Oct 16 15:04:45 EDT 2024
Wed Oct 16 15:04:45 EDT 2024
Wed Oct 16 15:04:45 GMT-04:00 2024
Mi. Okt. 16 15:04:45 EDT 2024
周三 10月 16 15:04:45 EDT 2024
Conclusion
As you can see we have a lot of flexibility for formatting values using the printf()
method in Java.
The examples used in this tutorial are available in my Github repository here.
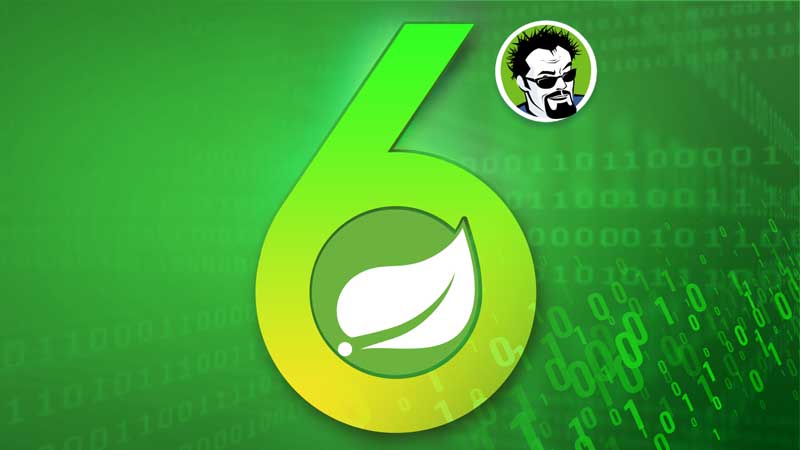
Spring Framework 6: Beginner to Guru
Checkout my best selling course on Spring Framework 6. This is the most comprehensive course you will find on Udemy. All things Spring!