Sorting ArrayLists in Java: A Practical Guide
3 CommentsLast Updated on October 22, 2024 by jt
Sorting an ArrayList is a common task in Java development. ArrayList
is one of the most commonly used collection classes of the Java Collection Framework. Java provides a number of different ways to sort ArrayLists.
In this post, we’ll explore the different ways to sort ArrayList
s, and review best practices you should keep in mind.
Why Sort an ArrayList?
Sorting an ArrayList
is needed when a collection of objects needs to be displayed in a particular order, or if you need to find a minimum or maximum value.
Using Collections.Sort() for Natural Ordering
Since ArrayList is part of the Java Collections Framework, we can use the Collections.sort() method. This works nicely on Wrapped Primitives (String, Integer, Long, etc), or objects which implement the Comparable
interface.
When you use a Natural Order Sort, it instructs the system to arrange elements in their “natural” sequence. For numbers, this means from the smallest to the largest. For strings, it refers to alphabetical order, starting from “A” to “Z”.
Sort ArrayList with Collections.sort()
In the example below, we take a list of 5 names and sort the list to alphabetical order.
import java.util.ArrayList;
import java.util.Collections;
public class CollectionSort
{
public static void main( String[] args )
{
ArrayList<String> list = new ArrayList<String>();
list.add("Michael Weston");
list.add("Fiona Glenanne");
list.add("Sam Axe");
list.add("Jesse Porter");
list.add("Madeline Westen");
System.out.printf("Before Sort : %s%n", list);
Collections.sort(list);
System.out.printf("After Sort : %s%n", list);
}
}
---------------output-----------------------
Before Sort : [Michael Weston, Fiona Glenanne, Sam Axe, Jesse Porter, Madeline Westen]
After Sort : [Fiona Glenanne, Jesse Porter, Madeline Westen, Michael Weston, Sam Axe]
The names are now in sorted alphabetical order. You can use this same method for sorting doubles, longs, and strings.
Reverse Sort ArrayList with Collections.sort()
The following example is the same as the previous, except we demonstrate how you can reverse the natural order of the sort with Collections.sort()
.
import java.util.ArrayList;
import java.util.Collections;
/**
* Created by jt, Spring Framework Guru.
*/
public class CollectionSortReverse {
public static void main( String[] args )
{
ArrayList<String> list = new ArrayList<String>();
list.add("Michael Weston");
list.add("Fiona Glenanne");
list.add("Sam Axe");
list.add("Jesse Porter");
list.add("Madeline Westen");
System.out.printf("Before Sort : %s%n", list);
Collections.sort(list, Collections.reverseOrder());
System.out.printf("After Sort : %s%n", list);
}
}
Sorting Objects with Custom Comparators
To help us sort objects, Collections.sort() can utilize a Comparator to allow us to specify custom sort criteria.
You can see for the output, we are using Java’s versatile printf() method.
Person Class
For our demonstration of using a Comparator, we’ll implement a simple Person POJO.
public static class Person {
private String firstName;
private String lastName;
public Person(String firstName, String lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
// getters and setters ommitted
}
Comparator Implementation
To create a Comparator, we need to provide an implementation of the java.util.Comparator
class.
In this example, we are comparing the firstName
property of our Person
POJO.
public static class CompareFirstName implements java.util.Comparator<Person> {
@Override
public int compare(Person o1, Person o2) {
return o1.getFirstName().compareTo(o2.getFirstName());
}
}
Collections.sort() with Comparator Example
In the following example, we create a list of 5 Person
objects, and use the Comparator
implementation to sort the list by firstName
.
public static void main( String[] args )
{
ArrayList<Person> list = new ArrayList<Person>();
list.add(new Person("Michael", "Weston"));
list.add(new Person("Fiona", "Glenanne"));
list.add(new Person("Sam", "Axe"));
list.add(new Person("Jesse", "Porter"));
list.add(new Person("Madeline", "Westen"));
System.out.printf("Before Sort : %s%n", list);
Collections.sort(list, new CompareFirstName());
System.out.printf("After Sort : %s%n", list);
}
Collections.sort() Lambda Example
We can also use a Java Lambda expression as the Comparator. In the following example we sort the list of 5 person objects by the lastName property.
public class CollectionSortLambda {
public static void main( String[] args )
{
ArrayList<Person> list = new ArrayList<Person>();
list.add(new Person("Michael", "Weston"));
list.add(new Person("Fiona", "Glenanne"));
list.add(new Person("Sam", "Axe"));
list.add(new Person("Jesse", "Porter"));
list.add(new Person("Madeline", "Westen"));
System.out.printf("Before Sort : %s%n", list);
Collections.sort(list, (p1, p2) -> p1.getLastName().compareTo(p2.getLastName()));
System.out.printf("After Sort : %s%n", list);
}
}
-----------------output-------------------
Before Sort : [{firstName='Michael', lastName='Weston'}, {firstName='Fiona', lastName='Glenanne'}, {firstName='Sam', lastName='Axe'}, {firstName='Jesse', lastName='Porter'}, {firstName='Madeline', lastName='Westen'}]
After Sort : [{firstName='Sam', lastName='Axe'}, {firstName='Fiona', lastName='Glenanne'}, {firstName='Jesse', lastName='Porter'}, {firstName='Madeline', lastName='Westen'}, {firstName='Michael', lastName='Weston'}]
Sort ArrayList with List sort() Method
The Java ArrayList
implements the List
interface, which provides a default sort(
) method. The List sort()
method functions similarly to the Collections.Sort()
method.
The following example shows:
- Sort List by Natural Order
- Sort List by Reverse Natural Order
- Sort List by Java Lambda expression
public static void main( String[] args )
{
ArrayList<String> list = new ArrayList<String>();
list.add("Michael Weston");
list.add("Fiona Glenanne");
list.add("Sam Axe");
list.add("Jesse Porter");
list.add("Madeline Westen");
System.out.printf("Before Sort : %s%n", list);
list.sort(Comparator.naturalOrder());
System.out.printf("After Natural Order Sort : %s%n", list);
list.sort(Comparator.reverseOrder());
System.out.printf("After Reverse Order Sort : %s%n", list);
list.sort((s1, s2) -> s1.split(" ")[1].compareTo(s2.split(" ")[1]));
System.out.printf("After Lambda Sort by Last Name : %s%n", list);
}
---------------output------------------------
Before Sort : [Michael Weston, Fiona Glenanne, Sam Axe, Jesse Porter, Madeline Westen]
After Natural Order Sort : [Fiona Glenanne, Jesse Porter, Madeline Westen, Michael Weston, Sam Axe]
After Reverse Order Sort : [Sam Axe, Michael Weston, Madeline Westen, Jesse Porter, Fiona Glenanne]
After Lambda Sort by Last Name : [Sam Axe, Fiona Glenanne, Jesse Porter, Madeline Westen, Michael Weston]
Sorting ArrayList with Java Streams
Java’s Stream
API also allows sorting using the sorted()
method. It can be particularly useful when you need to chain sorting with other operations like filtering.
Like the previous example, we can use the Java Stream API to:
- Sort List by Natural Order
- Sort List by Reverse Natural Order
- Sort List by Java Lambda expression
public static void main( String[] args ) {
ArrayList<String> list = new ArrayList<String>();
list.add("Michael Weston");
list.add("Fiona Glenanne");
list.add("Sam Axe");
list.add("Jesse Porter");
list.add("Madeline Westen");
System.out.printf("Before Sort : %s%n", list);
System.out.println("\nSorted with stream");
list.stream()
.sorted()
.forEach(System.out::println);
System.out.println("\nSorted with stream in reverse order");
list.stream()
.sorted(Comparator.reverseOrder())
.forEach(System.out::println);
System.out.println("\nSorted with stream by last name");
list.stream()
.sorted((s1, s2) -> s1.split(" ")[1].compareTo(s2.split(" ")[1]))
.forEach(System.out::println);
}
---------------------output-------------------------
Before Sort : [Michael Weston, Fiona Glenanne, Sam Axe, Jesse Porter, Madeline Westen]
Sorted with stream
Fiona Glenanne
Jesse Porter
Madeline Westen
Michael Weston
Sam Axe
Sorted with stream in reverse order
Sam Axe
Michael Weston
Madeline Westen
Jesse Porter
Fiona Glenanne
Sorted with stream by last name
Sam Axe
Fiona Glenanne
Jesse Porter
Madeline Westen
Michael Weston
Best Practices for Sorting
- Immutable Collections: If possible, consider using immutable collections when sorting data to avoid accidental modification of the original list
- Efficiency: Sorting a large list can be costly in terms of time (O(n log n)), avoid unnecessary sorting operations
- Null Handling: Ensure that your sorting logic can handle
null
values if they exist in your lists, or explicitly exclude them - Thread Safety: Be cautious when sorting lists in multi-threaded environments. If the
ArrayList
is accessed by multiple threads, consider usingsynchronizedList
or another thread-safe collection
Conclusion
Sorting ArrayList
objects is a common task that can be accomplished in several ways. We can sort ArrayLists using the simple Collections.sort()
method, or more advanced techniques using custom comparators, lambdas, and the Stream
API. By using these methods, you can efficiently manage data in your Java applications and ensure your lists are properly ordered based on your needs.
The source code for this post is available here in Github.
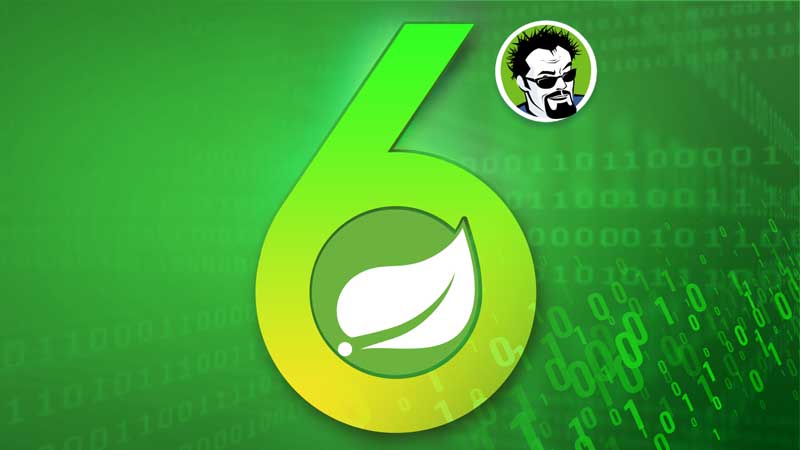
Spring Framework 6: Beginner to Guru
Checkout my best selling course on Spring Framework 6. This is the most comprehensive course you will find on Udemy. All things Spring!
MadhavaRao
Very good explanation about sorting arrayList.
Thank you John sharing your experience with us.
jt
Thanks!!