How To – The Java XOR Operator
1 CommentLast Updated on October 25, 2024 by jt
In this short tutorial we will look at using the Java XOR operator. We will discuss how the operator works, and how you can use it in your Java applications.
The XOR Operator
The XOR operator, also known as an exclusive OR operator is one of Java’s bitwise operators. The XOR operator is represented by the ^
(carrot) symbol.
The XOR Operator works with boolean and integer (int, long, byte) types only.
Logical XOR
When used with boolean values, the XOR functions as a logical operator.
Logical XOR Truth Table
When dealing with boolean values, the XOR operator will only return true if the operands are different.
A | B | A ^ B |
true | true | false |
true | false | true |
false | true | true |
false | false | false |
Logical XOR Example
The following Java statements:
boolean isSunny = true;
boolean isWarm = true;
System.out.println("Is it sunny and warm? " + (isSunny ^ isWarm));
Will produce the following output:
Is it sunny and warm? false
If we change the isSunny
variable to false
:
boolean isSunny = true;
boolean isWarm = false;
System.out.println("Is it sunny and warm? " + (isSunny ^ isWarm));
Will change the output to:
Is it sunny and warm? true
Bitwise XOR
When the XOR operator is used with integer values, it will perform a bitwise comparison.
Just as a refresher, a byte is composed of 8 bits. As a bitwise operator, the result is calculated from comparing each bit in the byte or bytes.
Bitwise XOR Truth Table
When comparing bytes, the Bitwise XOR operator applies the following logic.
A | B | A ^ B |
0 | 0 | 0 |
0 | 1 | 1 |
1 | 0 | 1 |
1 | 1 | 0 |
Bitwise XOR Examples
The following example in Java:
int f = 1; // 00000001
int g = 10; // 00001010
System.out.println("Result: " + (f ^ g)); // 00001011
Will produce:
Result: 11
To illustrate how the result was calculated, consider the following table.
1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | |
1 | 0 | 0 | 0 | 0 | 0 | 0 | 1 | 1 |
10 | 0 | 0 | 0 | 0 | 1 | 0 | 1 | 0 |
1 ^ 10 | 0 | 0 | 0 | 0 | 1 | 0 | 1 | 0 |
The XOR bitwise operation on each bit of the two values results in the binary value of 00001010
, which is the binary value of the decimal 11
.
At first glance you maybe thinking the XOR operator is doing a mathematical operation since 1 + 10 = 11
.
If you run this code, it could seem that way.
int i = 0;
int j = 0;
for (int k = 0; k < 15; k++) {
System.out.printf("values: %d ^ %d = %d | %d + %d = %d \n", i, j, i ^ j, i, j, i + j);
j++;
}
The results seem that XOR is doing a math operation:
values: 0 ^ 0 = 0 | 0 + 0 = 0
values: 0 ^ 1 = 1 | 0 + 1 = 1
values: 0 ^ 2 = 2 | 0 + 2 = 2
values: 0 ^ 3 = 3 | 0 + 3 = 3
values: 0 ^ 4 = 4 | 0 + 4 = 4
values: 0 ^ 5 = 5 | 0 + 5 = 5
values: 0 ^ 6 = 6 | 0 + 6 = 6
values: 0 ^ 7 = 7 | 0 + 7 = 7
values: 0 ^ 8 = 8 | 0 + 8 = 8
values: 0 ^ 9 = 9 | 0 + 9 = 9
values: 0 ^ 10 = 10 | 0 + 10 = 10
However, if we just change the starting value, we can see we get a much different result from the XOR operation.
int i = 5;
int j = 5;
for (int k = 0; k < 15; k++) {
System.out.printf("values: %d ^ %d = %d | %d + %d = %d \n", i, j, i ^ j, i, j, i + j);
j++;
}
values: 5 ^ 5 = 0 | 5 + 5 = 10
values: 5 ^ 6 = 3 | 5 + 6 = 11
values: 5 ^ 7 = 2 | 5 + 7 = 12
values: 5 ^ 8 = 13 | 5 + 8 = 13
values: 5 ^ 9 = 12 | 5 + 9 = 14
values: 5 ^ 10 = 15 | 5 + 10 = 15
values: 5 ^ 11 = 14 | 5 + 11 = 16
values: 5 ^ 12 = 9 | 5 + 12 = 17
values: 5 ^ 13 = 8 | 5 + 13 = 18
values: 5 ^ 14 = 11 | 5 + 14 = 19
values: 5 ^ 15 = 10 | 5 + 15 = 20
Conclusion
In this post we demonstrated the dual nature of the Java XOR operator. When applied to boolean values, the XOR operator functions as a logical comparison. When applied to integers, we explored how the XOR operator is doing a bitwise operation.
As always, you can find the source code for this post in my Github repository here.
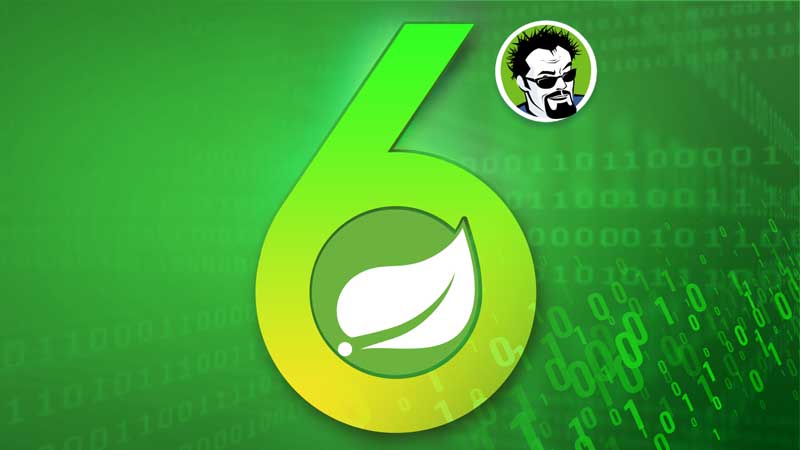
Spring Framework 6: Beginner to Guru
Checkout my best selling course on Spring Framework 6. This is the most comprehensive course you will find on Udemy. All things Spring!
Bala
pls change binary value of 1 . int f = 1; // 00000011 to int f = 1; // 00000001 in table also..