Introduction to Spring AI
0 CommentsLast Updated on October 14, 2024 by jt
Spring AI is the latest project from the Spring Team. Working with Large Language Models (LLMs) has traditionally been the domain of languages such as Python and Javascript. Spring AI is changing this and is enabling integration with generative AI with Java and Spring.
In this post, we will take an initial look at using Spring AI.
Spring AI Project
Dependencies
We will setup a very simple Spring Boot Application to demonstrate calling OpenAI with Spring AI.
Add the following dependencies to your Maven POM.
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.ai</groupId>
<artifactId>spring-ai-openai-spring-boot-starter</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
Spring AI Question and Answer Java Records
Add the following two Java records to your Spring Boot project.
Question.java
public record Question(String question) {
}
Answer.java
public record Answer(String answer) {
}
Spring AI Question Controller
We’ll use a Spring MVC Controller to allow RESTFul calls to our Spring AI Application. The OpenAIService
is defined in the next section.
This controller has one endpoint to allow us to post a question, and receive the response from OpenAI.
QuestionController.java
@RestController
public class QuestionController {
private final OpenAIService openAIService;
public QuestionController(OpenAIService openAIService) {
this.openAIService = openAIService;
}
@PostMapping("/ask")
public Answer askQuestion(@RequestBody Question question) {
return openAIService.getAnswer(question);
}
}
OpenAI Service
OpenAIService.java
public interface OpenAIService {
Answer getAnswer(Question question);
}
OpenAI Service Implementation
When Spring AI and OpenAI are on the classpath, Spring Boot will autoconfigure an instance of the Spring AI ChatModel
for use with OpenAI.
The Spring AI ChatModel
interface is a standard API for interacting with LLMs.
In the following implementation we ask Spring to autowire an instance of the ChatModel
into our service implementation.
We create a PromptTemplate
using the question string being passed into the getAnswer
method.
The call
method is used to invoke the call to OpenAI.
The ChatResponse
object contains a variety of metadata about result returned by the LLM.
In this case, we are only interested in the content of the response. The content is returned in the Java Answer
record, which is also the response provided in the Spring MVC controller.
OpenAIServiceImpl.java
@Service
public class OpenAIServiceImpl implements OpenAIService {
private final ChatModel chatModel;
public OpenAIServiceImpl(ChatModel chatModel) {
this.chatModel = chatModel;
}
@Override
public Answer getAnswer(Question question) {
PromptTemplate promptTemplate = new PromptTemplate(question.question());
Prompt prompt = promptTemplate.create();
ChatResponse response = chatModel.call(prompt);
return new Answer(response.getResult().getOutput().getContent());
}
}
OpenAI Configuration
To test this application, you will need an OpenAI API key. To obtain your API Key, you will need to create account with OpenAI and create an API key. You can setup your OpenAI account here.
You should treat the OpenAI API key as a secret, much like you would with a password. Clearly this is not something you should store plain text or save in GitHub.
The easiest way to manage the OpenAI API key is by using an environment variable.
In this example, we will use the environment variable OPENAI_API_KEY
to store the value of your OpenAI API key (sk-*******************
).
Add the line below to your application.properties file to bind the environment variable to the property Spring AI will use for the Spring Boot autoconfiguration of Spring AI.
Once this is set, we are ready to test our Spring AI application!
application.properties
#Pass API key as environment variable, e.g. -DOPENAI_API_KEY=your-api-key
spring.ai.openai.api-key=${OPENAI_API_KEY}
Testing the Spring AI Application
Start your Spring Boot application so that it is listening on port 8080 (the Spring Boot default).
We can start Postman and create a HTTP POST request to http://localhost:8080
with a JSON body of {"question" : "Tell me a Chuck Norris Joke."}

If everything is correct, you will see the following response from OpenAI.

And that’s it. You now have a Spring Boot application using Spring AI to interact with OpenAI!
The source code for this post is available here on Github.
If you’d like to learn about using StringTemplates with Spring AI, check out this post.
Spring AI: Beginner to Guru
If you wish to learn much more about Spring AI, check out my Udemy course Spring AI: Beginner to Guru!
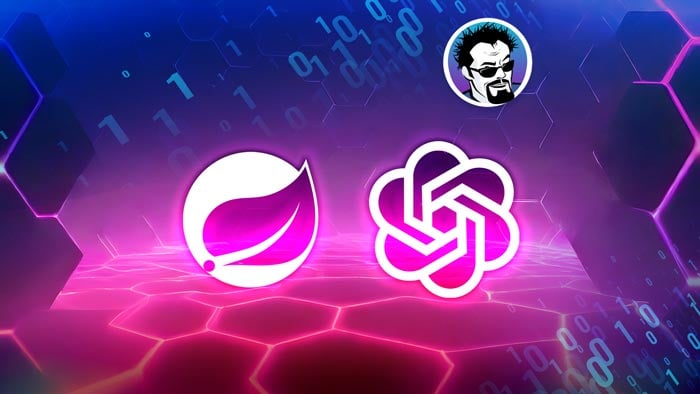