Hello World With Spring 4
0 CommentsLast Updated on June 16, 2019 by Simanta
This is a simple hello world example using Spring Framework 4. We will use Spring Boot and Spring Initializer to get things kicked off easily.
Video Tutorial
Follow this quick video tutorial to get a Spring Boot starter project.
Step by Step Instructions
Get Starter Project from Spring Initializer
Step One
- Go to the Spring Initializer website via this link.
- For our hello world example, all the defaults are fine.
- Click the Generate Button.
This will download a zip file containing a starter project to your hard drive.
Import Project Into IntelliJ
Step Two
Unzip the contents of the downloaded file to a working directory.
You now have a basic Spring project using Maven. The downloaded file contains the default Maven project structure. At the root of the directory is a pre-configured POM that contains a Spring Boot dependency. The Spring Boot dependency has child dependencies for Spring Core modules.
Step Three
Import project into your IDE. Our favorite IDE is IntelliJ.
- From IntelliJ go to File / New / Project from Existing Sources.
- Locate the file pom.xml in the root folder of the downloaded project and click OK.
- Click next from the Import from Maven screen.
- Select Maven Project, Click Next.
- Select the SDK to use. (You should select Java 1.8 or higher)
- Select name for the project. (Demo is fine for this)
- At this point the Maven project has been imported into the IntelliJ IDE.
Newsletter
Join the Spring Guru Newsletter!
Stay updated on the latest and greatest happening at springframework.guru.
[mc4wp_form]
Update Code
Step Four
Create a HelloWorld class which will print ‘Hello World’ to the console in Java.
@Component public class HelloWorld { public void sayHello(){ System.out.println("Hello World"); } }
Step Five
Update the main method to get an instance of the HelloWorld bean from the Spring Context.
@SpringBootApplication public class DemoApplication { public static void main(String[] args) { ApplicationContext ctx = SpringApplication.run(DemoApplication.class, args); HelloWorld helloWorld = (HelloWorld) ctx.getBean("helloWorld"); helloWorld.sayHello(); } }
Run Project – Say Hello
Step Six
Run the main method.
____ _ __ _ _ /\\ / ___'_ __ _ _(_)_ __ __ _ \ \ \ \ ( ( )\___ | '_ | '_| | '_ \/ _` | \ \ \ \ \\/ ___)| |_)| | | | | || (_| | ) ) ) ) ' |____| .__|_| |_|_| |_\__, | / / / / =========|_|==============|___/=/_/_/_/ :: Spring Boot :: (v1.2.2.RELEASE) 2015-03-11 10:25:18.981 INFO 7394 --- [ main] demo.DemoApplication : Starting DemoApplication on Johns-MacBook-Pro.local with PID 7394 (/Users/jt/src/springframework.guru/blog/hello-world-spring-4/demo/target/classes started by jt in /Users/jt/src/springframework.guru/blog/hello-world-spring-4/demo) 2015-03-11 10:25:19.050 INFO 7394 --- [ main] s.c.a.AnnotationConfigApplicationContext : Refreshing org.springframework.context.annotation.AnnotationConfigApplicationContext@3e57cd70: startup date [Wed Mar 11 10:25:19 EDT 2015]; root of context hierarchy 2015-03-11 10:25:20.143 INFO 7394 --- [ main] o.s.j.e.a.AnnotationMBeanExporter : Registering beans for JMX exposure on startup 2015-03-11 10:25:20.159 INFO 7394 --- [ main] demo.DemoApplication : Started DemoApplication in 1.517 seconds (JVM running for 2.092) Hello World 2015-03-11 10:25:20.162 INFO 7394 --- [ Thread-1] s.c.a.AnnotationConfigApplicationContext : Closing org.springframework.context.annotation.AnnotationConfigApplicationContext@3e57cd70: startup date [Wed Mar 11 10:25:19 EDT 2015]; root of context hierarchy 2015-03-11 10:25:20.164 INFO 7394 --- [ Thread-1] o.s.j.e.a.AnnotationMBeanExporter : Unregistering JMX-exposed beans on shutdown Process finished with exit code 0
Get The Code
Source Code
Want to Learn More About Spring?
Free Spring Framework Tutorial
Are you new to the Spring Framework? Checkout my completely free online tutorial for the Spring Framework!
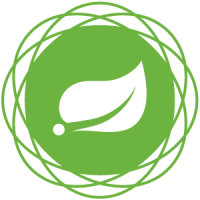
Get Access the Spring Framework Guru’s free Introduction to Spring Tutorial!
The first module of the Spring Framework Guru’s free Introduction to the Spring Framework tutorial is ready. Get access to the tutorial by clicking the link below.